How to find time difference in vb.net
VB .NET data types
VB .NET data types
There is no Variant data type in VB .NET. There are some other changes to
the traditional VB data types. A new Char type, which is an unsigned 16-bit
type, is used to store Unicode characters. The Decimal type is a 96-bit signed
Here are all the VB .NET data types:
Boolean System.Boolean 4 bytes True or False
Char System.Char 2 bytes 0–65535 (unsigned)
Byte System.Byte 1 byte 0–255 (unsigned)
Object System.Object 4 bytes Any Type
Date System.DateTime 8 bytes 01-Jan-0001 to
31-Dec-9999
Double System.Double 8 bytes +/–1.797E308
Decimal System.Decimal 12 bytes 28 digits
Integer System.Int16 2 bytes –32,768 to 32,767
System.Int32 4 bytes +/–2.147E9
Long System.Int64 8 bytes +/–9.223E18
Single System.Single 4 bytes +/–3.402E38
String System.String CharacterCount 2 billion
* 2 (plus 10 bytes) characters
Data Type Size in Bytes Description Type Byte 1 8-bit unsigned integer System.Byte Char 2 16-bit Unicode characters System.Char Integer 4 32-bit signed integer System.Int32 Double 8 64-bit floating point variable System.Double Long 8 64-bit signed integer System.Int64 Short 2 16-bit signed integer System.Int16 Single 4 32-bit floating point variable System.Single String Varies Non-Numeric Type System.String Date 8 System.Date Boolean 2 Non-Numeric Type System.Boolean Object 4 Non-Numeric Type System.Object Decimal 16 128-bit floating point variable System.Decimal
Adding a button to a form by code in vb.net
Assume that the user clicked a button asking to search for some information. You then create and display a TextBox for the user to enter the search criteria,and you also put a labelabove it describing the TextBox’s purpose
Converting Data Types in vb.net
Converting Data Types
VB .NET offers four ways to change one data type into another. The .ToString
method is designed to convert any numeric data type into a text string.
The second way to convert data is to use the traditional VB functions: CStr(),
CBool(), CByte(), CChar(), CShort(), CInt(), CLng(), CDate(), CDbl(),
CSng(), CDec(), and CObj(). Here is an example:
Dim s As String
Dim i As Integer = 1551
s = CStr(i)
MessageBox.Show(s)
The third way is to use the Convert method, like this:
Dim s As String
Dim i As Integer = 1551
s = Convert.ToString(i)
MessageBox.Show(s)
The fourth way uses the CType function, with this syntax:
Dim s As String
Dim i As Integer = 1551
s = CType(i, String)
MessageBox.Show(s)
Closing event in vb.net
Graphics features of vb.net
Graphics features of vb.net
There are many other methods you can use with a graphics object besides the DrawRectangle and FillRectangle methods illustrated in this example. Use DrawEllipse to draw circles and other round objects, and also try DrawLine, DrawPolygon, and others. The four arguments at the end of this line of code describe the horizontal (12) and vertical (15) point on the form where the upper-left corner of your rectangle is located, and the width (36) and height (45) of the rectangle: grObj.FillRectangle(brushBlue, 12, 15, 36, 45) The PSet and Point commands, which allowed you to manipulate individual pixels in earlier versions of VB, can be simulated by using the SetPixel or GetPixel methods of the System.Drawing Bitmap class. You can create a solid graphic (a square, circle, and so on) by simply using a brush to fill the area, without first using a pen to draw an outline
==========================================================================
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
'create graphics object
Dim grObj As System.Drawing.Graphics
grObj = Me.CreateGraphics
'create a red pen
Dim penRed As New System.Drawing.Pen(System.Drawing.Color.PaleVioletRed)
'make it fairly wide
penRed.Width = 9
'draw a square
grObj.DrawRectangle(penRed, 12, 12, 45, 45)
'create a brush (to fill the square)
Dim brushBlue As System.Drawing.Brush
brushBlue = New SolidBrush(Color.DarkBlue)
grObj.FillRectangle(brushBlue, 12, 12, 45, 45)
End Sub
=========================================================================
Array Search and Sort Methods in vb.net
Array Search and Sort Methods in vb.net
You can now have arrays search themselves or sort themselves. Here’s an example showing how to use both the sort and search methods of the Array object. The syntax for these two methods is as follows:
Array.Sort(myArray)
And
arrayIndex = Array.BinarySearch(myArray, “Name”)
To see this feature in action, put a Button and a TextBox control on a form and change the TextBox’s MultiLine property to True. Then enter the following
Search for “ArrayList” in vb.net
Reverses the order of the objects in the ArrayList in vb.net
how to fill an ArrayList with any number of duplicate objects in vb.net

How to add arraylist value to listbox in vb.net
This function clears out the ListBox, displays the array in the ListBox,
and then displays the count (the total number of items in the ArrayList) in
Try catch in vb.net
=================================================================
Try
'your code here
Catch ex As Exception
MessageBox.Show(ex.Message) 'if any error message show
End Try
================================================================
Try
Dim i As Int16 = "lk" 'for cheeking
Catch ex As Exception
MessageBox.Show(ex.Message) 'error message
End Try
=================================================================
How to format integer number to decimal number format in vb.net
How to show 1 with two decimal places (1.00)
How to add .00 to integer in vb.net
How to add 10 with .00 to get (10.00)
How to remove more than two decimal points
How to get 1.90001 to show with two decimal places (1.99)
---------------------------------------------------------------------------------------------
Txtamount.Text = Format(CType(Txtamount.Text, Double), "#0.00")
----------------------------------------------------------------------------------------------
How to remove decimal place in vb.net
How to get 1.90001 to show with two decimal places (1.99)
----------------------------------------------------------------------------------
Dim amount As Double = Math.Round(CType(Txtamount.Text, Double), 2)
------------------------------------------------------------------------------------------
Message box yes or no condition in vb.net
--------------------------------------------------------------------------------------------
If MessageBox.Show("Bill added successfully do u want to print the bill ", "", MessageBoxButtons.YesNo) = Windows.Forms.DialogResult.Yes Then
print() 'function for printing
End If
-----------------------------------------------------------------------------------------------
How to validate Coma in vb.net
-------------------------------------------------------------------------------------- Private Sub Txtname_KeyPress(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles Txtname.KeyPress
validation1(e)
End Sub
'function for coma validation
Public Sub validation1(ByVal e As System.Windows.Forms.KeyPressEventArgs)
Dim i As String = e.KeyChar.ToString()
if i = "," Then
e.Handled = True
End If
End Sub
------------------------------------------------------------------------------------
How to validate single quotes in vb.net
One of the most common problems in submitting vb forms to a database using access is the dreaded single quote. The database reads single quotes as part of the syntax of the access query and invariable produces an error. so we can avoid single quotes with this validation1 function
...........................................................................................................................................................
Private Sub Txtname_KeyPress(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyPressEventArgs) Handles Txtname.KeyPress
validation1(e)
End Sub
'function for single quotes validation
Public Sub validation1(ByVal e As System.Windows.Forms.KeyPressEventArgs)
Dim i As String = e.KeyChar.ToString()
If i = "'" Then ' for single quotes validation
e.Handled = True
End If
End Sub
---------------------------------------------------------------------------------------------
How to declare array in vb.net
with 10 elements indexed from X(0) up to X(9):
How to find current directory in vb.net
Download Qt software

![]() | |
---|---|
Screenshot [show] | |
Developer(s) | Qt Development Frameworks (formerly known as Trolltech) |
Stable release | 4.5.3 / 2009-10-01; 16 days ago |
Written in | C++ |
Operating system | Cross-platform |
Development status | Active |
Type | Widget toolkit |
License | GNU LGPL GNU GPL with Qt special exception Proprietary[1] |
Website | qt.nokia.com |
Download Visual Studio 2008 Service Pack 1

visual Studio 2008 delivers on Microsoft's vision of
How to make paging for grid in asp.net
Read function for asp.net
Read function for asp.net
Insert function for asp.net
{
int ret;
con.Open();
SqlCommand cmd = new SqlCommand(sav, con);
ret = cmd.ExecuteNonQuery();
con.Close();
return ret;
}
************************************************************************************
public void insert_function(string sav)
{
con.Open();
SqlCommand cmd = new SqlCommand(sav, con);
cmd.ExecuteNonQuery();
con.Close();
}
Stored Queries in access
click Queries under the Objects tab on the left. Now double click 'Create query in Design View'.
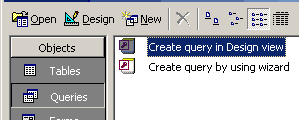
Two windows will open. Close the 'Show Tables' window by clicking the close button
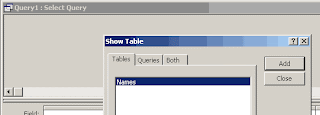
Now right click in the 'Query1 : Select Query' window and click 'SQL View' option as shown below.
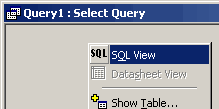
You should get a wide white window with something like following written on it's top left.
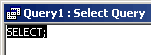
How to call Calculator in vb.net
----------------------------------------------------------------------------------------------------
Private Sub CalculatorToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles CalculaterToolStripMenuItem.Click
Dim q As New Process
q.StartInfo = New ProcessStartInfo("calc.exe")
q.Start()
q.WaitForExit()
End Sub
----------------------------------------------------------------------------------------------------
What are the difference between DDL, DML and DCL commands?
Data Definition Language (DDL) statements are used to define the database structure or schema. Some examples:
* CREATE - to create objects in the database
* ALTER - alters the structure of the database
* DROP - delete objects from the database
* TRUNCATE - remove all records from a table, including all spaces allocated for the records are removed
* COMMENT - add comments to the data dictionary
* RENAME - rename an object
DML
Data Manipulation Language (DML) statements are used for managing data within schema objects. Some examples:
* SELECT - retrieve data from the a database
* INSERT - insert data into a table
* UPDATE - updates existing data within a table
* DELETE - deletes all records from a table, the space for the records remain
* MERGE - UPSERT operation (insert or update)
* CALL - call a PL/SQL or Java subprogram
* EXPLAIN PLAN - explain access path to data
* LOCK TABLE - control concurrency
DCL
Data Control Language (DCL) statements. Some examples:
* GRANT - gives user's access privileges to database
* REVOKE - withdraw access privileges given with the GRANT command
TCL
Transaction Control (TCL) statements are used to manage the changes made by DML statements. It allows statements to be grouped together into logical transactions.
* COMMIT - save work done
* SAVEPOINT - identify a point in a transaction to which you can later roll back
* ROLLBACK - restore database to original since the last COMMIT
* SET TRANSACTION - Change transaction options like isolation level and what rollback segment to use
Email validation in VB.Net 2005
-------------------------------------------------------------------------------------------------
Imports System.Text.RegularExpressions
Public Sub email_val()
If Txtemail.Text <> "" Then
Dim rex As Match = Regex.Match(Trim(Txtemail.Text), "^([0-9a-zA-Z]([-.\w]*[0-9a-zA-Z])*@([0-9a-zA-Z][-\w]*[0-9a-zA-Z]\.)+[a-zA-Z]{2,3})$", RegexOptions.IgnoreCase)
If rex.Success = False Then
MessageBox.Show("Please Enter a valid Email-Address ", "Information", MessageBoxButtons.OK, MessageBoxIcon.Information)
Txtemail.Focus()
Exit Sub
End If
End If
End Sub
--------------------------------------------------------------------------------------------------
HOW TO FOCUS TEXTBOX TO LISTVIEW
--------------------------------------------------------------------------------------------------
Private Sub TextBox1_KeyDown(ByVal sender As System.Object, ByVal e As System.Windows.Forms.KeyEventArgs) Handles TextBox1.KeyDown
If ListView1.Items.Count > 0 Then
If e.KeyCode = Keys.Enter Or e.KeyCode = Keys.Down Then
ListView1.Focus()
ListView1.Items(0).Selected = True
End If
End If
End Sub
---------------------------------------------------------------------------------------------------
How To Remove Access Database Password
Removing the Database Password
You can remove the password you have set for a database. Once a password
is set, the choice in the Tools, Security menu choice becomes Unset
Database Password. You will be prompted for the password; it is case-sensitive.
After you remove the database password, anyone has access to the database.
Let's remove the database password you set for databasename.mdb
Removing a database password.
- Close databasename.mdb. Open databasename.mdb in Exclusive Mode.
- Enter dbpassword. Click OK
- Choose Tools, Security, Unset Database Password.
Notice how this command has a different name now that the database
has a password set on it. - In the Password text box, type dbpassword.
- Click OK to accept the password and close the dialog box.
- Let's test this change. Close the database.
- Open databasename.mdb. You didn't need to enter a
database password to open the database.